Developing web apps with Blazor technology has a lot of benefits. One of the biggest benefits is being able to use C# for both front-end and backend, simplifying the toolkit for creating rich web apps.
When you are using plain server-side rendered HTML, one would usually be required to use Javascript for making AJAX calls and load in new HTML. However, with Blazor you don’t have to anymore.
Add a timer thread
In order to periodically update the page contents, we will use a separate timer thread to periodically execute some logic.
For the sake of this example, we will use the default Blazor Server App project and alter the “counter” page to make it increase the value every second, without needing to click a button.
1. Create Blazor Server App
First, create a new Blazor Server App in Visual Studio:
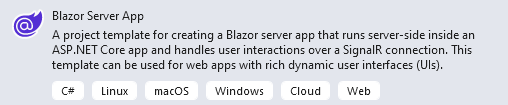
Afterwards, run the project in Debug Mode to make sure the default is working. You should see this page:
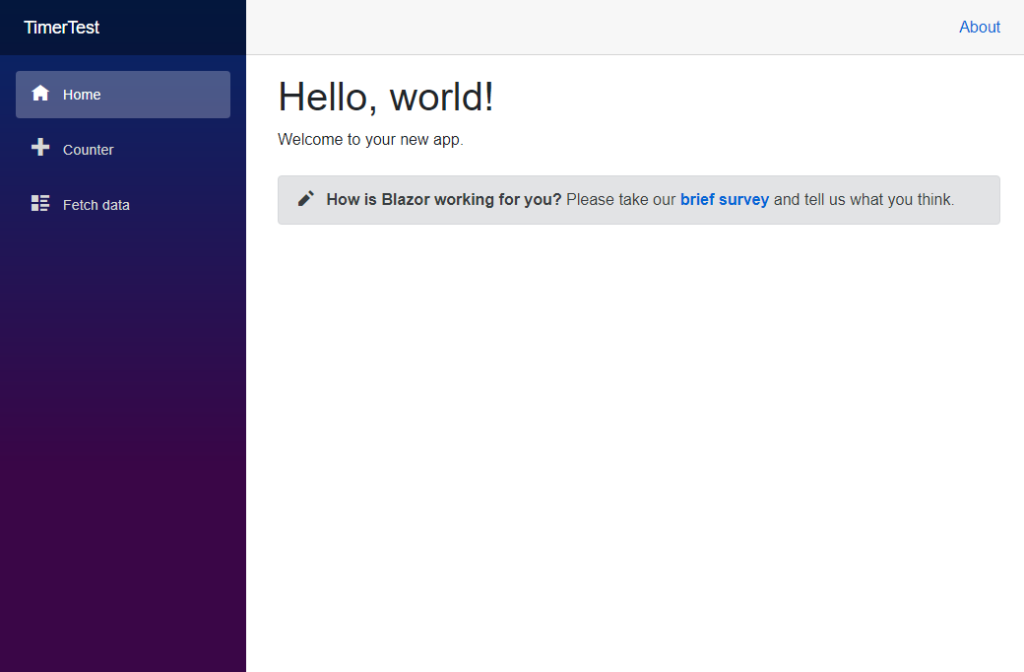
2. Open up the Counter page
Now, click on the “counter” page in the left menu. You should now see the following page. When clicking on the “Click me” button you will see the counter increase, like this:.
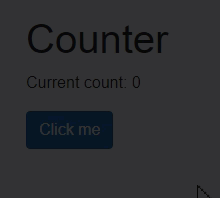
3. Add the async timer method
Now we will apply the background thread to increase the counter by itself. Steps:
- Open up the Pages/Counter.razor file in Visual Studio.
- Add a new method OnInitializedAsync(). This method will be automatically called when the page is opened.
protected override async Task OnInitializedAsync() { base.OnInitialized(); var timer = new System.Threading.Timer((_) => { InvokeAsync( async () => { // Add your update logic here IncrementCount(); // Update the UI StateHasChanged(); }); }, null, 0, 1000); }
- What the method above does is it creates a timer that is executed every second. Within the timer body we added an “InvokeAsync()” block where we call the already existing method called “IncrementCount()”. This is the same method that is called when the user presses the “Click me” button manually.
So in laymans terms: what this extra code does is it creates a timer that fires every second and presses the “count me” button for us. This should make it so that the counter on the page will automatically increment by 1 every second.
After adding the method above, the final Counter.Razor file should now look like this:
@page "/counter" <h1>Counter</h1> <p>Current count: @currentCount</p> <button class="btn btn-primary" @onclick="IncrementCount">Click me</button> @code { private int currentCount = 0; private void IncrementCount() { currentCount++; } protected override async Task OnInitializedAsync() { base.OnInitialized(); var timer = new System.Threading.Timer((_) => { InvokeAsync( async () => { // Add your update logic here IncrementCount(); // Update the UI StateHasChanged(); }); }, null, 0, 1000); } }
4. Try it out
Now run the project again in debug mode and open the “Counter” page. It will now automatically update the counter every second without needing to click the button.
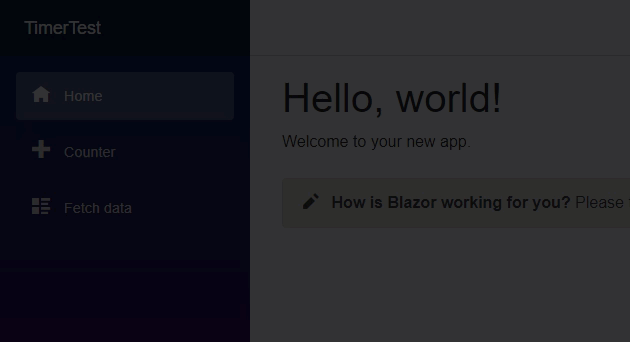
You can alter the code so instead of incrementing the counter it does other things, like periodically retrieve new information from a database.
Good luck!